Methods of Object Binding
Graphical objects Text, Label, Bitmap and Bitmap Label (OBJ_TEXT, OBJ_LABEL, OBJ_BITMAP and OBJ_BITMAP_LABEL) can have one of the 9 different ways of coordinate binding defined by the OBJPROP_ANCHOR property.
The following designations are used in the table:
- X/Y - coordinates of anchor points specified in pixels relative to a chart corner;
- Width/Height - objects have width and height. For "read only", the width and height values are calculated only once the object is rendered on chart;
- Date/Price - anchor point coordinates are specified using the date and price values;
- OBJPROP_CORNER - defines the chart corner relative to which the anchor point coordinates are specified. Can be one of the 4 values of the ENUM_BASE_CORNER enumeration;
- OBJPROP_ANCHOR - defines the anchor point in object itself and can be one of the 9 values of the ENUM_ANCHOR_POINT enumeration. Coordinates in pixels are specified from this very point to selected chart corner;
- OBJPROP_ANGLE - defines the object rotation angle counterclockwise.
The necessary variant can be specified using the function ObjectSetInteger(chart_handle, object_name, OBJPROP_ANCHOR, anchor_point_mode), where anchor_point_mode is one of the values of ENUM_ANCHOR_POINT.
ENUM_ANCHOR_POINT
|
|
ANCHOR_LEFT_UPPER
|
Anchor point at the upper left corner
|
ANCHOR_LEFT
|
Anchor point to the left in the center
|
ANCHOR_LEFT_LOWER
|
Anchor point at the lower left corner
|
ANCHOR_LOWER
|
Anchor point below in the center
|
ANCHOR_RIGHT_LOWER
|
Anchor point at the lower right corner
|
ANCHOR_RIGHT
|
Anchor point to the right in the center
|
ANCHOR_RIGHT_UPPER
|
Anchor point at the upper right corner
|
ANCHOR_UPPER
|
Anchor point above in the center
|
ANCHOR_CENTER
|
Anchor point strictly in the center of the object
|
The OBJ_BUTTON, OBJ_RECTANGLE_LABEL and OBJ_EDIT objects have a fixed anchor point in the upper left corner (ANCHOR_LEFT_UPPER).
Example:
void OnStart()
{
string text_name="my_OBJ_TEXT_object";
if(ObjectFind(0,text_name)<0)
{
Print("Object ",text_name," not found. Error code = ",GetLastError());
//--- Get the maximal price of the chart
double chart_max_price=ChartGetDouble(0,CHART_PRICE_MAX,0);
//--- Create object Label
ObjectCreate(0,text_name,OBJ_TEXT,0,TimeCurrent(),chart_max_price);
//--- Set color of the text
ObjectSetInteger(0,text_name,OBJPROP_COLOR,clrWhite);
//--- Set background color
ObjectSetInteger(0,text_name,OBJPROP_BGCOLOR,clrGreen);
//--- Set text for the Label object
ObjectSetString(0,text_name,OBJPROP_TEXT,TimeToString(TimeCurrent()));
//--- Set text font
ObjectSetString(0,text_name,OBJPROP_FONT,"Trebuchet MS");
//--- Set font size
ObjectSetInteger(0,text_name,OBJPROP_FONTSIZE,10);
//--- Bind to the upper right corner
ObjectSetInteger(0,text_name,OBJPROP_ANCHOR,ANCHOR_RIGHT_UPPER);
//--- Rotate 90 degrees counter-clockwise
ObjectSetDouble(0,text_name,OBJPROP_ANGLE,90);
//--- Forbid the selection of the object by mouse
ObjectSetInteger(0,text_name,OBJPROP_SELECTABLE,false);
//--- redraw object
ChartRedraw(0);
}
} |
Graphical objects Arrow (OBJ_ARROW) have only 2 ways of linking their coordinates. Identifiers are listed in ENUM_ARROW_ANCHOR.
ENUM_ARROW_ANCHOR
|
|
ANCHOR_TOP
|
Anchor on the top side
|
ANCHOR_BOTTOM
|
Anchor on the bottom side
|
Example:
#property strict
void OnStart()
{
//--- Auxiliary arrays
double Ups[],Downs[];
datetime Times[];
//--- Set the arrays as timeseries
ArraySetAsSeries(Ups,true);
ArraySetAsSeries(Downs,true);
ArraySetAsSeries(Times,true);
//--- Set Last error value to Zero
ResetLastError();
//--- Copy timeseries containing the opening bars of the last 1000 ones
int copied=CopyTime(NULL,0,0,1000,Times);
if(copied<=0)
{
Print("Unable to copy the Open Time of the last 1000 bars");
return;
}
//--- prepare the Ups[] and Downs[] arrays
ArrayResize(Ups,copied);
ArrayResize(Downs,copied);
//--- copy the values of iFractals indicator
for(int i=0;i<copied;i++)
{
Ups[i]=iFractals(NULL,0,MODE_UPPER,i);
Downs[i]=iFractals(NULL,0,MODE_LOWER,i);
}
//---
int upcounter=0,downcounter=0; // count there the number of arrows
bool created;// the result of attempts to create an object
for(int i=2;i<copied;i++)// Run through the values of the indicator iFractals
{
if(Ups[i]!=0)// Found the upper fractal
{
if(upcounter<10)// Create no more than 10 "Up" arrows
{
//--- Try to create an "Up" object
created=ObjectCreate(0,string(Times[i]),OBJ_ARROW_THUMB_UP,0,Times[i],Ups[i]);
if(created)// If set up - let's make tuning for it
{
//--- Point anchor is below in order not to cover bar
ObjectSetInteger(0,string(Times[i]),OBJPROP_ANCHOR,ANCHOR_BOTTOM);
//--- Final touch - painted
ObjectSetInteger(0,string(Times[i]),OBJPROP_COLOR,clrBlue);
upcounter++;
}
}
}
if(Downs[i]!=0)// Found a lower fractal
{
if(downcounter<10)// Create no more than 10 arrows "Down"
{
//--- Try to create an object "Down"
created=ObjectCreate(0,string(Times[i]),OBJ_ARROW_THUMB_DOWN,0,Times[i],Downs[i]);
if(created)// If set up - let's make tuning for it
{
//--- Point anchor is above in order not to cover bar
ObjectSetInteger(0,string(Times[i]),OBJPROP_ANCHOR,ANCHOR_TOP);
//--- Final touch - painted
ObjectSetInteger(0,string(Times[i]),OBJPROP_COLOR,clrRed);
downcounter++;
}
}
}
}
} |
After the script execution the chart will look like in this figure.
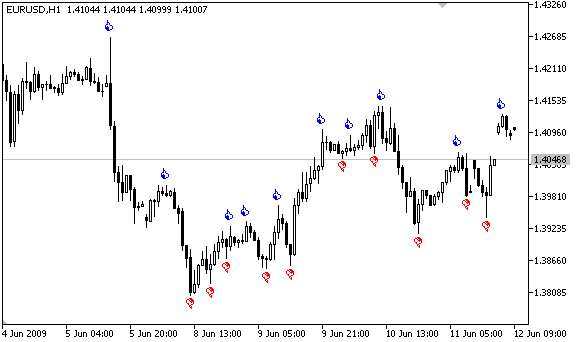
|